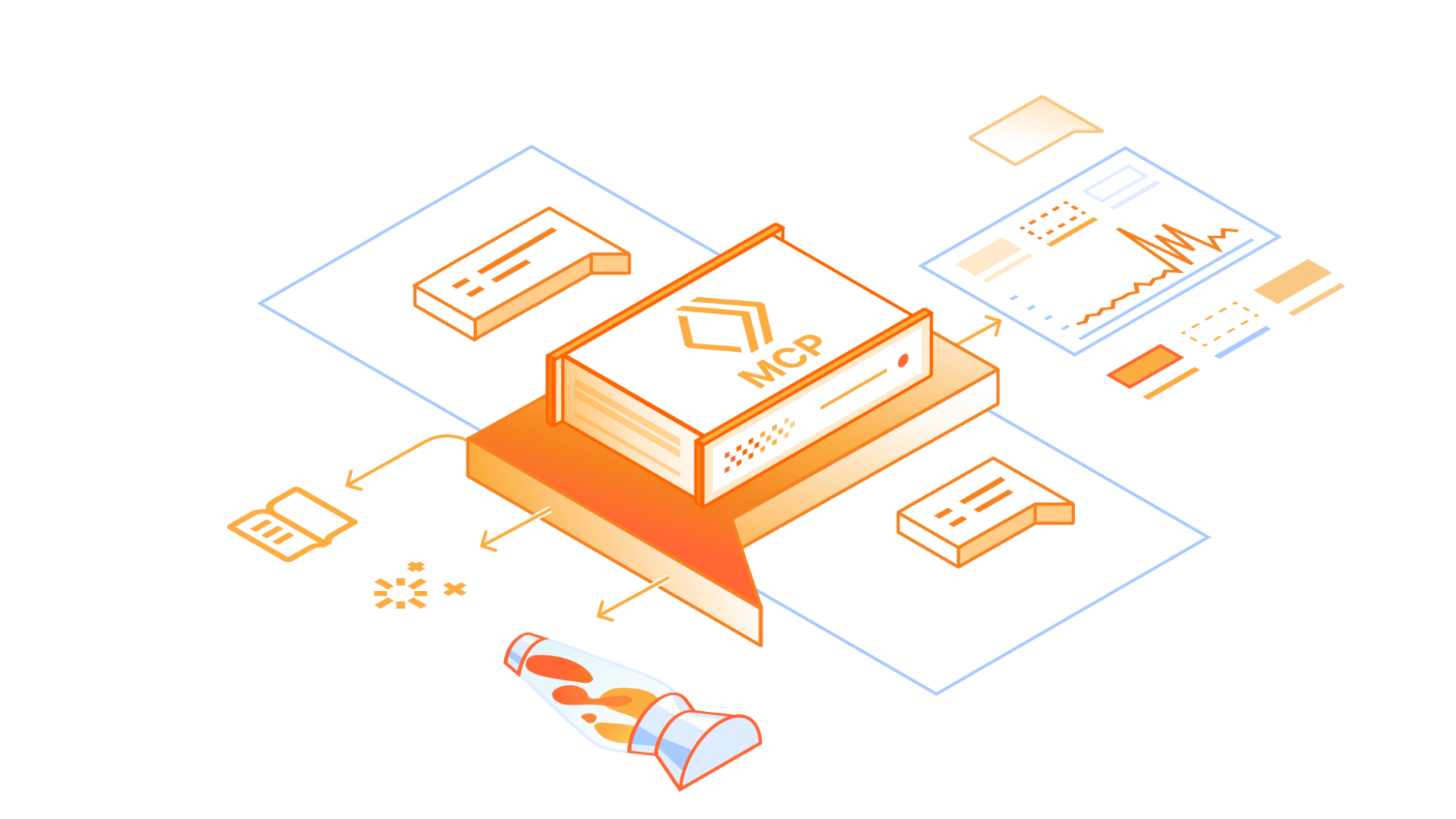
Unleashing Creativity and Efficiency with MCP: The Future of AI-Assisted Development
In today’s fast-evolving tech landscape, developers face mounting pressure to deliver high-quality software faster than ever before. Between juggling complex codebases, integrating external data sources, and keeping up with the latest tools, the demands can feel overwhelming. What if there were a way to empower your AI assistant to seamlessly fetch code from GitHub, query a database, or scour the web—all without leaving your coding environment? That’s where the Model Context Protocol (MCP) comes in, a transformative framework that’s redefining how developers and AI collaborate.
You’re debugging a tricky issue late at night, and instead of manually scouring documentation or switching between apps, your AI assistant instantly retrieves the latest library version, creates a GitHub issue, and suggests a fix—all in seconds. This isn’t a distant dream; it’s the reality MCP enables. In this blog post, we’ll unpack what MCP is, guide you through using it with tools like Cursor AI, explore real-world scenarios where it shines, and look ahead to its exciting future. Whether you’re a solo coder or part of a large team, MCP can elevate your workflow to new heights

What is MCP? A Universal Bridge for AI and Data
At its heart, MCP is an open-source standard designed to connect AI systems to the vast array of data sources developers rely on daily—think GitHub repositories, relational databases, APIs, or even web search engines. Developed by Anthropic, MCP eliminates the need for custom, one-off integrations by providing a unified way for AI tools to access and interact with external information. It’s like giving your AI assistant a master key to unlock data from anywhere, making it smarter and more versatile.
So how does it work? MCP operates through two key components:
- MCP Hosts: These are the AI interfaces you interact with, such as Cursor AI. The host is the “brain” that processes your commands and leverages external data.
- MCP Servers: These act as the “connectors,” linking the host to specific data sources. Each server exposes unique capabilities—whether it’s pulling files from a repository, querying a database, or performing a web search.

The Model Context Protocol (MCP) is a standardized framework that connects AI agents to external tools and data sources.
Similar to how a USB-C simplifies device connections to your computer, MCP streamlines how AI models interact with your data, tools, and services. In this context, your laptop serves as the MCP host.
This interplay between hosts and servers creates a standardized ecosystem where AI can tap into diverse resources without developers needing to reinvent the wheel for every new integration. For instance, instead of writing a bespoke script to fetch GitHub data, MCP provides a ready-made server that handles it for you. This standardization, detailed in resources like Model Context Protocol’s Introduction, is what makes MCP a game-changer.
But MCP isn’t just about convenience—it’s about power. By breaking down data silos, it enables AI to operate with richer context, leading to more accurate responses and sophisticated automation. Imagine an AI that not only understands your code but also pulls in real-time documentation or team discussions from Slack to inform its suggestions. That’s the promise of MCP.
Don’t see a server for your specific use case? No problem—you can create your own using tools like the Python MCP SDK or Typescript MCP SDK. This flexibility ensures MCP adapts to your unique needs, whether you’re connecting to a niche API or a custom database.
Getting Started with MCP and Cursor AI
Ready to harness MCP in your own projects? Setting it up with Cursor AI is simpler than you might think, and once it’s running, the possibilities are endless.
In this example, we’ll create an MCP server that:
- Fetches the contents of a file from a specified GitHub repository.
- Creates a new issue in the repository when instructed.
This server will use the GitHub API via the PyGitHub library and expose these capabilities to Cursor AI through MCP.
Prerequisites
Before we start, ensure you have:
- Python 3.8+ installed on your machine.
- uv installed. See: https://docs.astral.sh/uv/getting-started/installation/
- A GitHub account and a repository to test with (e.g., yourusername/test-repo).
- A GitHub Personal Access Token with repo scope (generate one at GitHub Settings > Developer Settings > Personal Access Tokens).

- Cursor AI installed and running.
Step 1: Set Up Your Environment with uv
Create a Project Directory:
1mkdir github-mcp-server-uv
2cd github-mcp-server-uv
Initialize a uv Project: Run this to create a pyproject.toml file and set up a virtual environment:
1uv init
This generates a basic pyproject.toml like:
1[project]
2name = "github-mcp-server-uv"
3version = "0.1.0"
4description = "MCP server for GitHub integration"
5dependencies = []
Add Dependencies: Use uv to add PyGitHub and aiohttp:
1uv add pygithub aiohttp
This updates pyproject.toml to include:
1[project]
2name = "github-mcp-server-uv"
3version = "0.1.0"
4description = "MCP server for GitHub integration"
5dependencies = [
6 "pygithub>=2.3.0",
7 "aiohttp>=3.9.0",
8]
uv also installs these into a local .venv folder.
Store Your GitHub Token: For security, set it as an environment variable:
1export GITHUB_TOKEN="your-token-here"
Step 2: Write the MCP Server Script
Create a file called github_mcp_server.py in your project directory and add this code:
1import os
2import json
3from github import Github
4from aiohttp import web
5import asyncio
6
7# Configuration
8GITHUB_TOKEN = os.getenv("GITHUB_TOKEN") # Get token from environment
9PORT = 8080 # Port for the MCP server
10
11# Initialize GitHub client
12github_client = Github(GITHUB_TOKEN)
13
14# Define MCP capabilities
15CAPABILITIES = {
16 "capabilities": [
17 {
18 "name": "fetch_file",
19 "description": "Fetches the contents of a file from a GitHub repository",
20 "parameters": {
21 "repo": {"type": "string", "description": "Repository name (e.g., username/repo)"},
22 "path": {"type": "string", "description": "Path to the file in the repo"}
23 }
24 },
25 {
26 "name": "create_issue",
27 "description": "Creates a new issue in a GitHub repository",
28 "parameters": {
29 "repo": {"type": "string", "description": "Repository name (e.g., username/repo)"},
30 "title": {"type": "string", "description": "Title of the issue"},
31 "body": {"type": "string", "description": "Body text of the issue"}
32 }
33 }
34 ]
35}
36
37# Handle MCP capability advertisement
38async def advertise_capabilities(request):
39 return web.Response(text=json.dumps(CAPABILITIES), content_type="application/json")
40
41# Handle MCP tool execution
42async def execute_tool(request):
43 try:
44 data = await request.json()
45 tool_name = data.get("name")
46 params = data.get("parameters", {})
47
48 if tool_name == "fetch_file":
49 repo_name = params.get("repo")
50 file_path = params.get("path")
51 if not repo_name or not file_path:
52 return web.Response(text=json.dumps({"error": "Missing repo or path"}), status=400)
53
54 repo = github_client.get_repo(repo_name)
55 file_content = repo.get_contents(file_path).decoded_content.decode("utf-8")
56 return web.Response(text=json.dumps({"content": file_content}), content_type="application/json")
57
58 elif tool_name == "create_issue":
59 repo_name = params.get("repo")
60 title = params.get("title")
61 body = params.get("body")
62 if not repo_name or not title:
63 return web.Response(text=json.dumps({"error": "Missing repo or title"}), status=400)
64
65 repo = github_client.get_repo(repo_name)
66 issue = repo.create_issue(title=title, body=body)
67 return web.Response(text=json.dumps({"issue_url": issue.html_url}), content_type="application/json")
68
69 return web.Response(text=json.dumps({"error": "Unknown tool"}), status=400)
70 except Exception as e:
71 return web.Response(text=json.dumps({"error": str(e)}), status=500)
72
73# Set up the aiohttp server
74app = web.Application()
75app.router.add_get("/capabilities", advertise_capabilities)
76app.router.add_post("/execute", execute_tool)
77
78# Start the server
79if __name__ == "__main__":
80 print(f"Starting MCP GitHub Server on port {PORT} with uv...")
81 web.run_app(app, port=PORT)
Step 3: Run the MCP Server with uv
1uv run python github_mcp_server.py
It should show
1Starting MCP GitHub Server on port 8080 with uv...
Verify It Works:
You’ll see the JSON response with fetch_file and create_issue. Great stuff!
1> curl http://localhost:8080/capabilities
2{"capabilities": [{"name": "fetch_file", "description": "Fetches the contents of a file from a GitHub repository", "parameters": {"repo": {"type": "string", "description": "Repository name (e.g., username/repo)"}, "path": {"type": "string", "description": "Path to the file in the repo"}}}, {"name": "create_issue", "description": "Creates a new issue in a GitHub repository", "parameters": {"repo": {"type": "string", "description": "Repository name (e.g., username/repo)"}, "title": {"type": "string", "description": "Title of the issue"}, "body": {"type": "string", "description": "Body text of the issue"}}}]}
Step 4: Integrate with Cursor AI
Open Cursor AI: Launch the app.
Add the MCP Server:
- Go to Settings > MCP Servers.
- Click “Add New MCP Server.”
- Name it (e.g., “GitHub MCP with uv”).
- Set the command to: uv run python /path/to/github_mcp_server.py (use the full path to your script, e.g., /home/user/github-mcp-server-uv/github_mcp_server.py).
- Save it.
Test Commands:
- “Fetch the contents of README.md from yourusername/test-repo.”
- “Create an issue in yourusername/test-repo titled ‘Test Issue’ with body ‘Created via MCP!’” Cursor AI will execute these via the server, returning file contents or an issue URL.
Real-World Scenarios: MCP’s Versatility Unleashed
MCP isn’t just a theoretical tool—it’s a practical powerhouse with applications across countless development tasks. Let’s dive into some detailed scenarios where MCP shines, showcasing how it can save time, boost productivity, and inspire creativity.
Streamlining GitHub Workflows
Managing a GitHub repository can feel like herding cats—tracking issues, reviewing pull requests, and syncing code across teams takes effort. MCP simplifies this by automating repetitive tasks.
Imagine you’re working on a feature and spot a bug. Rather than pausing to log into GitHub, you tell Cursor AI: “File a bug report for this crash in [repository URL], including the stack trace.” The GitHub MCP server springs into action, crafting an issue with the error details and linking it back to your codebase. Within moments, you’ve got a URL to the new issue, keeping your workflow uninterrupted. This seamless integration, as demonstrated in egghead.io’s MCP Guide, ensures nothing slips through the cracks.
For larger teams, MCP can even automate pull request reviews or notify contributors of updates, turning a chaotic process into a well-oiled machine.
Crafting Data-Driven Insights
Data is the lifeblood of many applications, but extracting it often involves tedious manual queries. MCP changes that by letting your AI assistant handle database interactions effortlessly.
Suppose you’re building an e-commerce dashboard and need sales stats. You ask Cursor AI: “Show me total revenue by product category for Q3.” Connected to your database via an MCP server, the AI runs the query, aggregates the data, and presents it in a clean format—no SQL expertise required. This capability, highlighted in Semantic Kernel’s MCP Integration Guide, is a boon for developers who need quick insights without diving into backend code.
Beyond reports, MCP can automate data imports, updates, or even trigger alerts based on specific conditions, making it a versatile tool for data-heavy projects.
Keeping Up with Real-Time Web Insights
In a field where technologies evolve daily, staying current is a challenge. MCP servers like Brave Search bring the web into your editor, delivering real-time answers without breaking your focus.
Say you’re integrating a new framework and need clarification: “What’s the best way to handle routing in Next.js 14?” Cursor AI, using a web search MCP server, scours the latest blog posts, docs, and forums, returning a concise summary with links to dive deeper. As noted in Medium’s MCP Tools Post, this keeps you in the loop without the distraction of browser tabs.
This is especially handy for troubleshooting obscure errors or researching best practices, ensuring your decisions are informed by the latest knowledge.
Simplifying File Management
File operations might seem mundane, but they’re a constant in development—creating configs, updating docs, or organizing assets. MCP turns these chores into one-liners.
For example, you’re setting up a new project and need a config file: “Generate a package.json with Express and TypeScript dependencies.” The AI, via an MCP server with file system access, creates the file, populates it with the right JSON, and saves it in your project directory. This automation, supported by servers in GitHub’s MCP Collection, saves time and reduces errors from manual edits.
Need to bulk-rename files or sync local changes with a remote repo? MCP can handle that too, making it a Swiss Army knife for project maintenance.
Bridging Third-Party Services
Modern apps often rely on external APIs—think payment gateways, messaging platforms, or weather services. MCP makes integrating these a breeze.
Imagine you’re building a chatbot and want to notify users via Telegram. You say: “Send ‘Project update: v1.0 live!’ to [chat ID] on Telegram.” An MCP server configured for the Telegram API authenticates, sends the message, and confirms delivery—all without you touching the API docs. This use case, explored in Portkey’s MCP Blog, showcases how MCP extends AI’s reach into the broader digital ecosystem.
From triggering CI/CD pipelines to posting Slack updates, MCP’s API integrations open up a world of automation possibilities.
Securing Your MCP Setup
With great power comes great responsibility, and MCP’s access to sensitive data demands careful attention to security. Here’s how to keep your setup safe and efficient:
- Lock Down Access: Use authentication mechanisms like OAuth or API tokens for servers connecting to services like GitHub. Limit permissions to only what’s necessary—don’t give full repo access if you just need issue tracking.
- Protect Data in Transit: Enable HTTPS or other encryption protocols for server communications, especially when handling private data like customer records.
- Optimize Performance: Monitor server resource usage to prevent slowdowns. A lightweight, well-tuned server ensures your AI stays snappy.
- Stay Updated: Regularly pull the latest server versions from GitHub to patch vulnerabilities and gain new features.
Joining the MCP Community
MCP isn’t a solo endeavor—it thrives on a vibrant community of developers pushing its boundaries. Here’s where to connect and learn:
- GitHub Hub: The Model Context Protocol GitHub is your go-to for code, docs, and server examples.
- Forums: Engage with peers on the Cursor Community Forum to troubleshoot or brainstorm new ideas.
- Learning Resources: Dive into tutorials on Medium or egghead.io for hands-on guidance.
Contributing a server or sharing a tip can also amplify your impact in this growing ecosystem.
The Horizon: MCP’s Role in Tomorrow’s Development
MCP is just getting started. As AI models grow more advanced, MCP will evolve to support richer interactions—think multi-agent systems collaborating on a project or AI proactively suggesting optimizations based on real-time repo analysis. The future might bring servers for emerging platforms, deeper integration with cloud services, or even voice-driven MCP commands.
For developers, embracing MCP now is an investment in tomorrow. It’s not just about automating today’s tasks—it’s about building a foundation for the next leap in AI-driven innovation, where coding becomes a partnership between human creativity and machine precision.
Wrapping Up: Why MCP Matters
The Model Context Protocol is more than a tool—it’s a paradigm shift. By connecting AI to the tools and data developers already use, it transforms workflows from fragmented to fluid, from manual to magical. Whether you’re streamlining GitHub tasks, pulling live data, or weaving in third-party services, MCP delivers efficiency and flexibility in spades.
So, take the plunge. Set up an MCP server, experiment with Cursor AI, and see how it reshapes your day-to-day coding. The future of development is here—and with MCP, you’re ready to lead the charge.